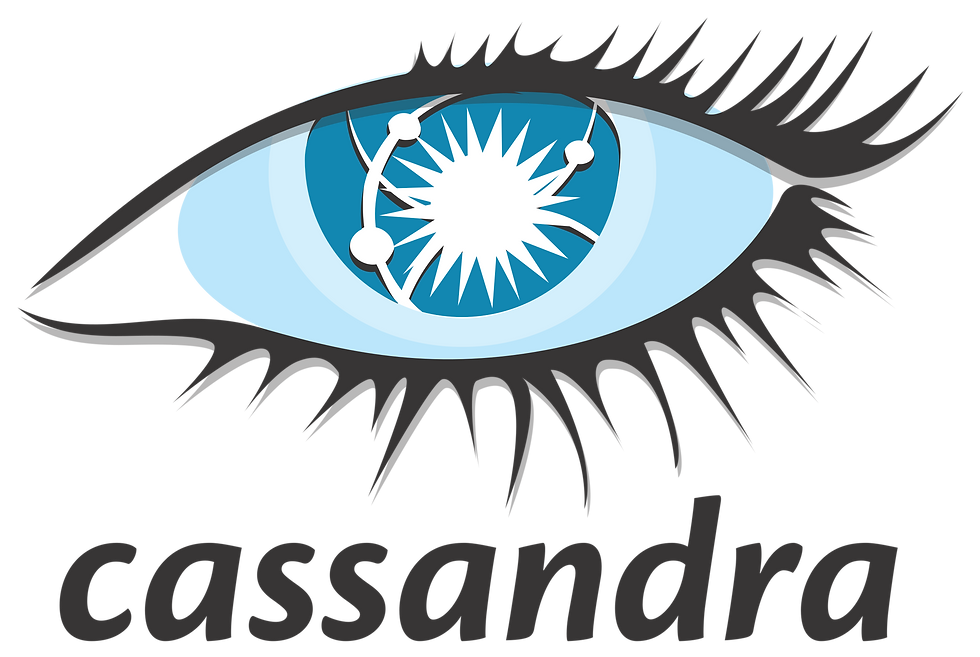
Introduction:
In this post, we'll look at how to perform CRUD (Create, Read, Update, and Delete) operations with Cassandra in a Spring Boot application. We'll cover the basic setup of a Spring Boot application, including the creation of a RESTful web service endpoint that communicates with a Cassandra database. We'll use a three-layer architecture, consisting of a Controller layer, a Service layer, and a Repository layer.
By the end of this post, you'll have a basic understanding of how to create, read, update, and delete data in a Cassandra database using Spring Boot. You'll also see how to map Cassandra data to Java objects using the Cassandra Mapping API provided by Spring Data Cassandra.
Controller :
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class UserController {
@Autowired
private UserService userService;
@PostMapping("/user")
public void addUser(@RequestBody User user) {
userService.addUser(user);
}
@GetMapping("/user")
public Iterable<User> getAllUsers() {
return userService.getAllUsers();
}
}
Service :
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
public void addUser(User user) {
userRepository.save(user);
}
public Iterable<User> getAllUsers() {
return userRepository.findAll();
}
}
Repository:
import org.springframework.data.cassandra.repository.CassandraRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface UserRepository extends CassandraRepository<User, Integer> {
}
Model:
import org.springframework.data.cassandra.core.mapping.PrimaryKey;
import org.springframework.data.cassandra.core.mapping.Table;
@Table
public class User {
@PrimaryKey
private int id;
private String name;
// getters and setters
}
Explanation:
The UserController class is a RESTful web service endpoint that maps HTTP requests to methods in the UserService class.
The UserService class implements business logic and communicates with the UserRepository class to persist data to and retrieve data from Cassandra.
The UserRepository class extends CassandraRepository, which provides basic CRUD operations for Cassandra.
The User class is a simple POJO (Plain Old Java Object) that represents a user in Cassandra. It has a primary key id and a name.
Note: This example assumes that you have set up a Cassandra cluster and created a keyspace and table to store user data.
Kommentarer